Registry Pattern
Purpose
The purpose of the design pattern
To implement a central storage for objects often used throughout the application, is typically implemented using an abstract class with only static methods (or using the Singleton pattern).
UML
UML design pattern diagram
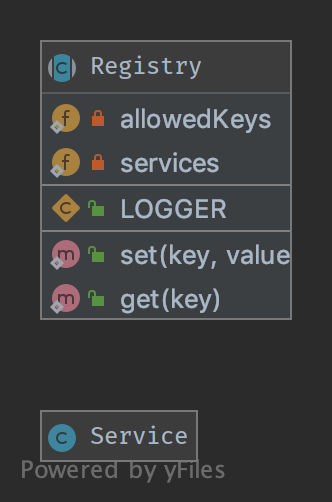
Code
Code snippets
Registry
Essentially gives the ability to create a registry of services via a static getter (parameter type hinted to an interface) and setter, which can be used in whichever class extends it.
namespace DesignPatterns\Structural\Registry;
use InvalidArgumentException;
abstract class Registry
{
public const LOGGER = 'logger';
/**
* @var Service[]
*/
private static array $services = [];
private static array $allowedKeys = [
self::LOGGER,
];
final public static function set(string $key, Service $value)
{
if (!in_array($key, self::$allowedKeys)) {
throw new InvalidArgumentException('Invalid key given');
}
self::$services[$key] = $value;
}
final public static function get(string $key): Service
{
if (!in_array($key, self::$allowedKeys) || !isset(self::$services[$key])) {
throw new InvalidArgumentException('Invalid key given');
}
return self::$services[$key];
}
}
Ready to bring your vision to life?
We believe in excellence, empathy, integrity, and transparency throughout the process. Our goal is to build fast, responsive websites that not only perform but also reflect your values and vision.