Purpose
The purpose of the design pattern
To write code that is easy readable just like sentences in a natural language (like English).
Examples
Examples of how the design pattern can be used
- A query builder which works something like that example class below.
- PHPUnit uses fluent interfaces to build mock objects.
UML
UML design pattern diagram
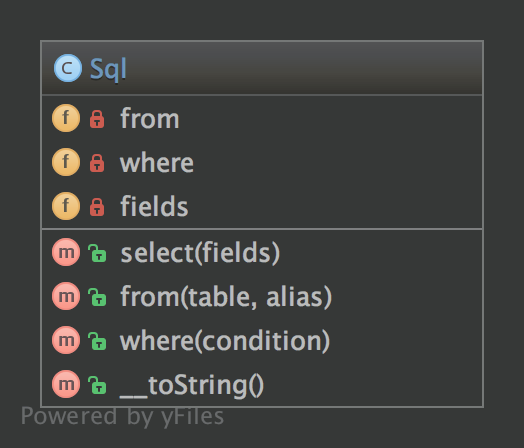
Code
Code snippets
Fluent Interface
This class is a Fluent Interface and reads like English.
Called something likeā¦
$query = new Sql;
echo $query->select(['stuff'])->from('somewhere')->where('id = 2');
$query = new Sql;
echo $query->select(['stuff'])->from('somewhere')->where('id = 2');
namespace DesignPatterns\Structural\FluentInterface;
class Sql implements \Stringable
{
private array $fields = [];
private array $from = [];
private array $where = [];
public function select(array $fields): Sql
{
$this->fields = $fields;
return $this;
}
public function from(string $table, string $alias): Sql
{
$this->from[] = $table . ' AS ' . $alias;
return $this;
}
public function where(string $condition): Sql
{
$this->where[] = $condition;
return $this;
}
public function __toString(): string
{
return sprintf(
'SELECT %s FROM %s WHERE %s',
join(', ', $this->fields),
join(', ', $this->from),
join(' AND ', $this->where)
);
}
}