Purpose
The purpose of the design pattern
To have only one instance of this object in the application that will handle all calls.
THIS IS CONSIDERED TO BE AN ANTI-PATTERN! FOR BETTER TESTABILITY AND MAINTAINABILITY USE DEPENDENCY INJECTION!
Examples
Examples of how the design pattern can be used
- DB Connector
- Logger
- Lock file for the application (there is only one in the filesystem ...)
UML
UML design pattern diagram
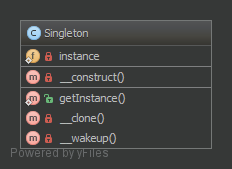
Code
Code snippets
Singleton
With a Singleton you’re not allowed to create multiple instances. The instance is retrieved via lazy initialisation. i.e Singleton::getInstance(). If the instance has already been created, that is returned, otherwise it is created and returned.
namespace DesignPatterns\Creational\Singleton;
use Exception;
final class Singleton
{
private static ?Singleton $instance = null;
/**
* gets the instance via lazy initialization (created on first usage)
*/
public static function getInstance(): Singleton
{
if (self::$instance === null) {
self::$instance = new self();
}
return self::$instance;
}
/**
* is not allowed to call from outside to prevent from creating multiple instances,
* to use the singleton, you have to obtain the instance from Singleton::getInstance() instead
*/
private function __construct()
{
}
/**
* prevent the instance from being cloned (which would create a second instance of it)
*/
private function __clone()
{
}
/**
* prevent from being unserialized (which would create a second instance of it)
*/
public function __wakeup()
{
throw new Exception("Cannot unserialize singleton");
}
}