Purpose
The purpose of the design pattern
SimpleFactory is a simple factory pattern.
It differs from the static factory because it is not static. Therefore, you can have multiple factories, differently parameterised, you can subclass it and you can mock it.
It has some advantages over a Static Factory but a Static Factory closes classes for modification, this technically doesn’t (if you needed to change the Simple Factory, you’d be adjusting the class its being called from).
Examples
Examples of how the design pattern can be used
UML
UML design pattern diagram
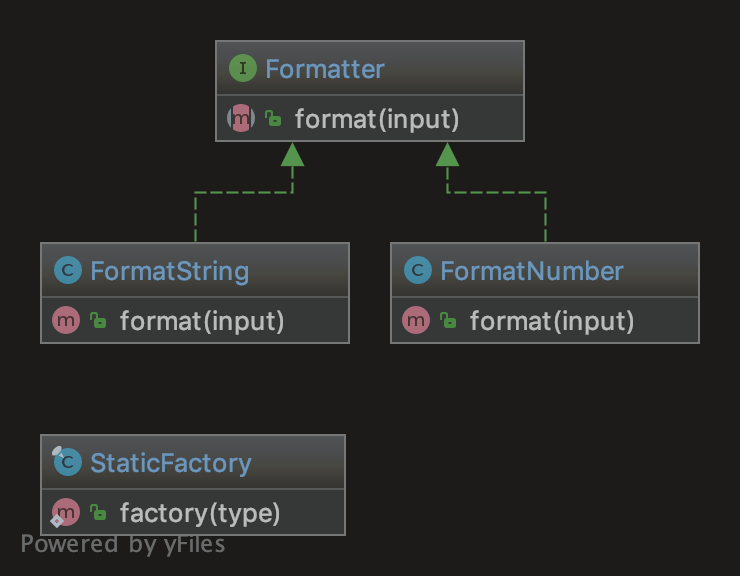
Code
Code snippets
Bicycle
Bicycle concrete class.
namespace DesignPatterns\Creational\SimpleFactory;
class Bicycle
{
public function driveTo(string $destination)
{
}
}
SimpleFactory
Defer to the SimpleFactory to return an instance of Bicycle. i.e:
container(SimpleFactory)->createBicycle();
namespace DesignPatterns\Creational\SimpleFactory;
class SimpleFactory
{
public function createBicycle(): Bicycle
{
return new Bicycle();
}
}