My primary focus over the last few years has been back-end development, however I've been brushing up on React in my spare time. I wanted to create tests (like I've writing tests for back-end functionality).
UI Testing, also known as GUI Testing is basically a mechanism meant to test the aspects of any software that a user will come into contact with. I came across 'Testing Library'...
The @testing-library family of packages helps you test UI components in a user-centric way. It currently has support for React, Vue, Native, Angular and many more.
Ever struggled to know which tests to write? Testing playground suggests tests - just install the Chrome or Firefox extension.
The following tests if the component renders and the Counter can increment and decrement (by simulating a click event):
import { fireEvent, render, screen } from '@testing-library/react';
import Counter from '../Counter';
import userEvent from '@testing-library/user-event';
test('renders the Counter component', () => {
render(<Counter title="My Counter" />);
const titleElement = screen.getByText(/My Counter/i);
expect(titleElement).toBeInTheDocument();
const countElement = screen.getByText(/Count: 0/i);
expect(countElement).toBeInTheDocument();
});
test('renders the Counter component using selectors', () => {
const { container } = render(<Counter title="My Counter" />);
const titleElement = container.querySelector('#title');
expect(titleElement.textContent).toContain('My Counter');
const countElement = container.querySelector('#count');
expect(countElement.textContent).toContain('Count: 0');
});
test('increments the counter', () => {
render(<Counter title="My Counter" />);
// screen.debug();
const incrementButton = screen.getByRole('button', {
name: /increment/i,
});
expect(incrementButton).toBeInTheDocument();
userEvent.click(incrementButton);
const countElement = screen.getByText(/Count: 1/i);
expect(countElement).toBeInTheDocument();
});
test('decrements the counter', () => {
render(<Counter title="My Counter" />);
// screen.debug();
const decrementButton = screen.getByRole('button', {
name: /decrement/i,
});
expect(decrementButton).toBeInTheDocument();
userEvent.click(decrementButton);
const countElement = screen.getByText(/Count: -1/i);
expect(countElement).toBeInTheDocument();
});
The React Testing Library is a very light-weight solution for testing React components.
npm install --save-dev @testing-library/react
Best practice is to create a tests directory and create a test version of your React component.
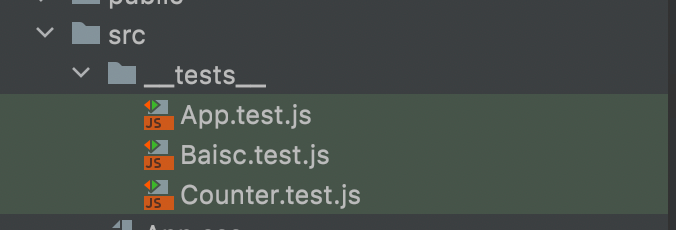
These will be picked up when the tests run. To run the test suite, run:
npm test
Whenever, a test is changed the test suite should re-run. Output will typically look something like this:
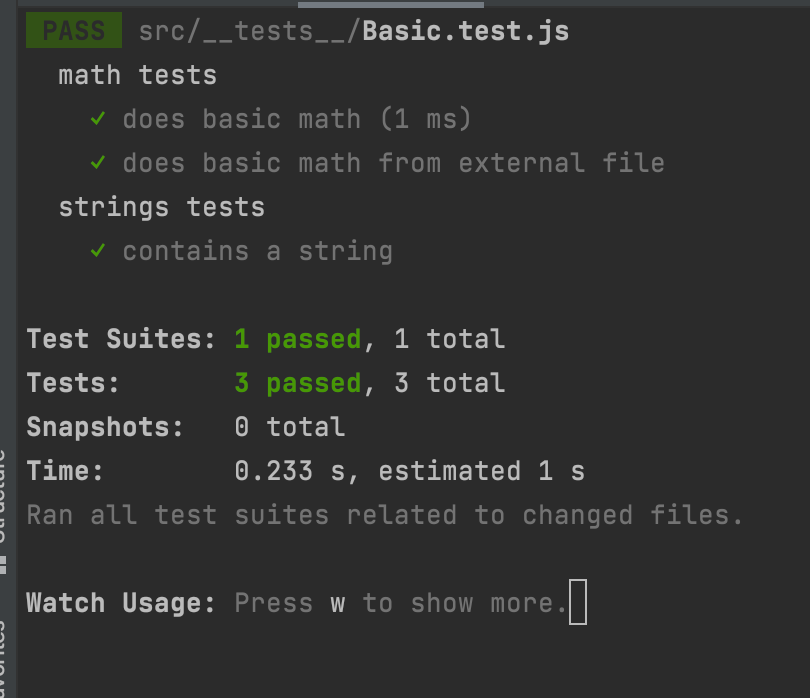
Basic Test Example
import { add, subtract, multiply } from '../Math.js';
describe('math tests', () => {
it('does basic math', () => {
expect(3 + 2).toBe(5);
expect(3 * 2).toBe(6);
expect(Math.sqrt(36)).toBe(6);
});
it('does basic math from external file', () => {
expect(add(3, 2)).toBe(5);
expect(multiply(3, 2)).toBe(6);
expect(subtract(3, 2)).toBe(1);
});
});